HOW DO I DO THAT IN MATLAB SERIES?
In this series, I am answering questions that students have asked me about MATLAB. Most of the questions relate to a mathematical procedure.
Contents
TOPIC
How do I conduct polynomial interpolation for given data.
SUMMARY
Language : Matlab 2010a; Authors : Autar Kaw and Sri Harsha Garapati; Mfile available at Last Revised : January 23, 2012; Abstract: This program shows you how to perform polynomial interpolation in MATLAB
clc
clear all
clf
INTRODUCTION
disp('ABSTRACT') disp(' This program shows you how to perform polynomial interpolation') disp(' ') disp('AUTHOR') disp(' Autar Kaw and Sri Harsha Garapati of http://autarkaw.wordpress.com') disp(' ') disp('MFILE SOURCE') disp(' http://numericalmethods.eng.usf.edu/blog/interpolation_polynomial_sec002_blog.m') disp(' ') disp('LAST REVISED') disp(' January 23, 2012') disp(' ')
ABSTRACT This program shows you how to perform polynomial interpolation AUTHOR Autar Kaw and Sri Harsha Garapati of http://autarkaw.wordpress.com MFILE SOURCE http://numericalmethods.eng.usf.edu/blog/interpolation_polynomial_sec002_blog.m LAST REVISED January 23, 2012
INPUTS
% Inputs assuming % that three points are given xd = [1 4 8]; % x data points yd = [2 5 7]; % y data points xval= 4.5; % predict y at this x value
DISPLAYING INPUTS
disp(' ') disp('INPUTS') % Creating a matrix to print input data as a table data=[xd;yd]'; disp(' X Y ') % Printing the input data as a table disp(data) % Printing the input value of X at which Y is to be predicted fprintf(' Predict the value of y when x is %g\n\n',xval)
INPUTS X Y 1 2 4 5 8 7 Predict the value of y when x is 4.5
THE CODE
% n returns the number of data points n =length(xd); % order of interpolation model or =n-1; % conducting polynomial interpolation coef=polyfit(xd,yd,or); % predicting y with the chosen interpolation model ypre=polyval(coef,xval); % setting up domain for plotting xp =[min(xd):0.1:max(xd)]; % evaluating over domain xp for plotting the interpolation function yp =polyval(coef,xp); % creating symbolic variable X to display the output function syms x % defining the function as a string fun =char(vpa(coef(1)*(x)+coef(2),5));
DISPLAYING OUTPUTS AND PLOTS
disp(' ') disp('OUTPUTS') % printing the predicted value of y fprintf(' Predicted value of y is %g\n',ypre) % printing the interpolation function fprintf(' The interpolation model is:\n %s\n\n',fun) disp(' See figure(1)') % Telling Matlab to name the figure as Figure-1 figure(1) % Matlab command so that it does hold on to all the plots hold on % plotting the data points plot(xd,yd,'rs','MarkerSize',10) % plotting the interpolation function plot(xp,yp,'k-.','LineWidth',2) % plotting the predicted data point value plot(xval,ypre,'bo','MarkerSize',10) % Title of the Plot title('Interpolation Model for Data Points') % Legend to distunguish different plots legend('Given Points','Interpolation Model','Predicted Point',0) % Labeling the X-axis xlabel('x') % Labeling the Y-Axis ylabel('y') % Matlab command to exit the hold-on option hold off
OUTPUTS Predicted value of y is 5.375 The interpolation model is: 1.3571 - 0.071429*x See figure(1)
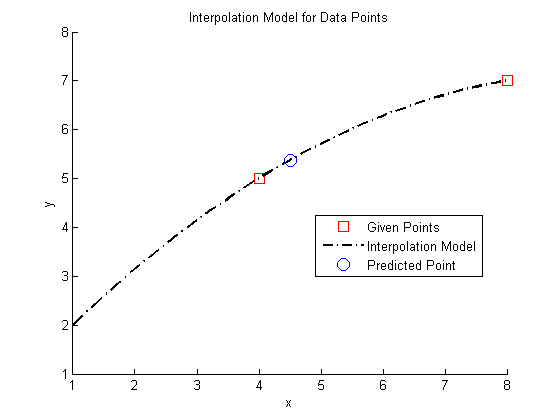